I started using Docker very recently with my open-source projects especially for local development and was amazed at how effective and efficient it is. I also used Docker to deploy my wordpress website/blog on Hetzner and it literally costs me just $8/month (can go down to $4 if you use a slightly less powerful server).
So if you are looking to dockerize your Laravel application you could use the yml file below to get started.
This is going to be super easy. Stay tuned!
We are going to look at setting up Docker containers for the following services and structuring our Laravel app.
- PostgreSQL DB
- Nginx
- php-fpm
- Running tests
- npm
- Supervisord for background jobs
- Laravel scheduler for cron jobs
This list is pretty much what I use for my projects.
Lets first create our project folder, you can name this anything but this is what we’d be committing to our git account.
I use a Mac and I have a folder called dockerapps created in the below path
(base) madhu@Madhukars-MacBook-Air dockerapps % pwd
/Users/madhu/Documents/dockerapps
(base) madhu@Madhukars-MacBook-Air dockerapps %
I am now going to create my project named “laravel_docker”
(base) madhu@Madhukars-MacBook-Air dockerapps % mkdir laravel_docker
(base) madhu@Madhukars-MacBook-Air dockerapps % ls
laravel_docker myblog seasonsurvey
(base) madhu@Madhukars-MacBook-Air dockerapps %
Since this is the file I am going to commit to git, I will initialize a git repo in it
(base) madhu@Madhukars-MacBook-Air dockerapps % cd laravel_docker
(base) madhu@Madhukars-MacBook-Air laravel_docker % git init
Initialized empty Git repository in /Users/madhu/Documents/dockerapps/laravel_docker/.git/
Now lets create a laravel project called “src” inside laravel_docker directory, this is where we will create our laravel application code will reside
(base) madhu@Madhukars-MacBook-Air laravel_docker % mkdir src
(base) madhu@Madhukars-MacBook-Air laravel_docker % cd src
(base) madhu@Madhukars-MacBook-Air src %
I will create a jetstream project but you can go with whatever you want.
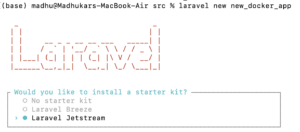
This is how your source directory looks like
(base) madhu@Madhukars-MacBook-Air laravel_docker % cd src
(base) madhu@Madhukars-MacBook-Air src % pwd
/Users/madhu/Documents/dockerapps/laravel_docker/src
(base) madhu@Madhukars-MacBook-Air src % ls
README.md bootstrap config node_modules phpunit.xml resources tailwind.config.js vite.config.js
app composer.json database package-lock.json postcss.config.js routes tests
artisan composer.lock jsconfig.json package.json public storage vendor
(base) madhu@Madhukars-MacBook-Air src %
Now that you have the application, lets start dockerizing the app.
Got to “laravel_docker” folder (whatever you named your project), it will look like so
(base) madhu@Madhukars-MacBook-Air laravel_docker % ls
src
(base) madhu@Madhukars-MacBook-Air laravel_docker %
Create a file called docker-compose.yml file
inside the file, copy and paste the below content
version: '3.8'
networks:
seasonsurvey:
name: seasonsurvey
services:
postgres:
image: postgres:14.12
container_name: postgres
restart: always
networks:
- seasonsurvey
ports:
- 5433:5432
volumes:
- ./postgresql/data:/var/lib/postgresql/data
environment:
- POSTGRES_DB=seasonsurvey
- POSTGRES_USER=postgres
- POSTGRES_PASSWORD=password
php:
build:
context: .
dockerfile: php.dockerfile
container_name: php
restart: always
volumes:
- ./src:/var/www/html
networks:
- seasonsurvey
nginx:
build:
context: .
dockerfile: nginx.dockerfile
container_name: nginx
restart: always
depends_on:
- php
- postgres
networks:
- seasonsurvey
ports:
- 80:80
volumes:
- ./src:/var/www/html
composer:
image: composer:latest
container_name: composer
volumes:
- ./src:/var/www/html
working_dir: /var/www/html
networks:
- seasonsurvey
llama31:
image: ilopezluna/llama3.1:0.3.11-8b
container_name: llama
networks:
- seasonsurvey
ports:
- 11435:11434
artisan:
build:
context: .
dockerfile: php.dockerfile
container_name: artisan
volumes:
- ./src:/var/www/html
working_dir: /var/www/html
entrypoint: ["php", "artisan"]
networks:
- seasonsurvey
npm:
image: node:current-alpine
container_name: npm
ports:
- 5173:5173
volumes:
- ./src:/var/www/html
working_dir: /var/www/html
entrypoint: ['npm']
networks:
- seasonsurvey
runtests:
build:
context: .
dockerfile: php.dockerfile
container_name: runtests
volumes:
- ./src:/var/www/html
working_dir: /var/www/html
entrypoint: ["php", "artisan", "test"]
networks:
- seasonsurvey
scheduler:
build:
context: .
dockerfile: php.dockerfile
container_name: scheduler
restart: always
volumes:
- ./src:/var/www/html
working_dir: /var/www/html
entrypoint: ["php", "artisan", "schedule:work"]
networks:
- seasonsurvey
supervisord:
build:
context: .
dockerfile: supervisord.dockerfile
container_name: supervisord
restart: always
depends_on:
- nginx
- php
- postgres
- scheduler
volumes:
- ./src:/var/www/html
working_dir: /var/www/html
networks:
- seasonsurvey
Now,
docker-compose up -d nginx supervisord
On your browser
http://localhost
And you are done!